HelpDesk SDK
Introduction
App Widgets are web applications loaded inside the HelpDesk App. They live in iframes and can interact and communicate with the HelpDesk App. Read about their possible locations in the App locations guide. The HelpDesk SDK is currently the primary way to develop your own widgets and can be used in a basic or advanced way.
Use cases
Widgets are primary elements of the HelpDesk user interface. You can use them to:
- automate your processes and workflows.
- integrate external online services or applications.
- customize your views, reports, or payment methods.
- translate your messages or tickets.
Before you start
From a technical point of view, widgets are regular web applications. For that reason, you need to have some knowledge of HTML, CSS, and JavaScript to build your own widget.
Bear in mind that widgets are front-end elements of the HelpDesk App. If your intention is to build a server-side app, we encourage you to check out the HelpDesk API.
Getting started
Follow the instructions to create a basic HelpDesk Widget. Before you start, make sure you have an account.
- Go to the Apps section in Developer Console.
- Click Create new app + and give your app a name.
- Decide which product you want to build your app for.
- If you do not have a HelpDesk account, you will be asked to activate it.
- Go to Building blocks and add a new HelpDesk Widgets block.
- Enter the Widget source URL, which is the address of your application.
- Choose the widget placement. It allows you to decide where exactly in the HelpDesk App you want to display your integration. Currently, the possible locations are the Details section, Fullscreen Apps (main menu), or App Settings. Read more about app locations.
- Additionally, you can configure your app's short decription and add an icon for better visibility in the HelpDesk App. You can do that in the Display details tab.
- Go to the Private installation tab and install the app on your account.
After the successful installation, you'll see the widget in your HelpDesk App.
Installing the SDK
You can download the SDK directly from NPM as an ES6 or CommonJS module.
Install the SDK from NPM...
INSTALL THE SDK FROM NPM
npm install @livechat/helpdesk-sdk
...then import the ES6 module...
IMPORT THE ES6 MODULE
import * as HelpDesk from "@livechat/helpdesk-sdk";
...or require the CommonJS.
REQUIRE THE COMMONJS
const HelpDesk = require("@livechat/helpdesk-sdk");
You can also use the UMD build of the SDK directly in the browser.
You can also use the UMD build:
USE UMD BUILD
<script src="https://unpkg.com/@livechat/helpdesk-sdk@1.0.3/dist/helpdesk-sdk.umd.min.js"></script>
<script>
HelpDesk.createDetailsWidget().then(function(widget) {
// do something with widget
});
</script>
Basic use
The specific usage of the HelpDesk SDK depends on the type of widget you want to create. The currently available widget types are the following:
All widgets created with the HelpDesk SDK share a common interface, which lets you listen for the events happening in the HelpDesk App.
Supposing that widget
is a widget instance:
function handleEvent(event) {
// perform logic when event happens,
// you can also use the data attached to the event
}
// Subscribe to event
widget.on("event", handleEvent);
// Unsubscribe from event
widget.off("event", handleEvent);
Each widget type offers a different set of events that you can listen for.
Details widgets
If you want to use your app in the Details section, you should use the createDetailsWidget
function. It returns a promise resolving to a details widget instance.
DETAILS WIDGETS
import { createDetailsWidget } from "@livechat/helpdesk-sdk";
createDetailsWidget().then(widget => {
// build your logic around the widget
});
Events
ticket_info
Emitted when an agent opens a conversation within tickets, or when an agents selects the ticket. The handler will get the ticket object as an argument.
Listen for the ticket changes:
ticket_info
widget.on("ticket_info", ticketInfo => {
// read the ticket object
});
The ticket object will have the following shape:
Property | Description |
---|---|
id | Unique object identifier |
shortId | Short ticket ID |
createdBy | Creator identifier |
updatedAt | Time of last modification |
updatedBy | Modification author identifier |
lastMessageAt | Time of last public message |
events | Ticket events |
requester | Ticket requester |
status | Ticket status: open , pending , onhold , solved , closed |
spam | Spam details |
subject | Ticket subject |
source | Ticket source |
tagIds | List of ticket tags |
teamIds | Teams that can access the ticket |
assignment | Team / agent assignment |
ratingRequestSent | A rating request has been sent |
rating | Ticket rating |
ccs | List of people in the loop |
priority | -10 : low, 0 : medium, 10 : high, 20 : urgent |
followers | List of ticket followers (agents) |
childTickets | Merged ticket reference |
parentTicket | Parent ticket reference |
customFields | Object with custom fields values, where key is custom fields apiKey |
ticket_details_section_button_click
Emitted when you click a button located in a section in Ticket Details. The handler gets the following payload:
Property | Description |
---|---|
buttonId | The id of the button taken from the section definition |
React to button clicks within Ticket Details widgets
ticket_details_section_button_click
widget.on("ticket_details_section_button_click", ({ buttonId }) => {
// perform an action when the button is clicked
});
Methods
Get the ticket info
If you want to access the current ticket, you should use the getTicketInfo
method.
Get the current ticket info
GET THE TICKET INFO
const ticketInfo = widget.getTicketInfo();
The returned profile will be an object identical to the one emitted by the tickets_info
event. It can also be null
if no info was recorded.
Modify Ticket Details widget's state
If you configured a Ticket Details widget, you can modify its section state using the modifySection
method. The method accepts the section state definition as its only parameter, and returns a promise.
You can look up the component types to see how to create the state definition.
The title
of a given section has to match the one specified in the Opening state. Otherwise, the section won't change. Also, the HelpDesk App ignores the commands without valid section definitions. Make sure that the definition you're sending is correct.
Modify the Ticket Details widget's state
MODIFY THE TICKET DETAILS WIDGET STATE
widget
.modifySection({
title: "My widget",
components: [
{
type: "title",
data: {
value: "The title will be modified"
}
}
]
})
.then(() => {
// the widget should be modified now
});
Fullscreen widgets
If you want to connect a Fullscreen widget to the HelpDesk App, you should use the createFullscreenWidget
function. It returns a promise resolving to a Fullscreen widget instance.
FULLSCREEN WIDGETS
import { createFullscreenWidget } from "@livechat/helpdesk-sdk";
createFullscreenWidget().then(widget => {
// build your logic around the widget
});
Events
This widget currently does not support any events.
Methods
Set notification badge
If you want to notify Agents there’s something important inside the widget, use the setNotificationBadge
method.
SET NOTIFICATION BADGE
widget.setNotificationBadge(26);
It displays a notification badge on top of your app’s icon:
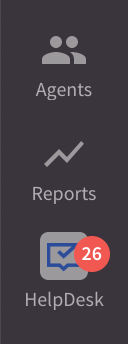
If you want to remove the badge, invoke the method with the null
parameter.
REMOVE NOTIFICATION BADGE
widget.setNotificationBadge(null);
Make sure Agents can dismiss the notification to avoid cluttered UI.
Advanced use
Developing your own widget
If you're building your own widget and you need to interact with the HelpDesk App, be sure to use the HelpDesk SDK.
Accessing HelpDesk data
You can leverage the OAuth 2.1 authorization flow to use HelpDesk API. Head to Sign in with LiveChat docs for more information.
Layout and Styling
If you're using React, you can use the LiveChat Design System, which is a component library used directly in the LiveChat user interface.
Hosting the widget
You can host your widget locally or on a dedicated server. The hosted content has to be served over the HTTPS Protocol.
During development, you can use a self-signed certificate for localhost
or upload your widget to an SSL-enabled host. You can also leverage bundlers like Webpack to use an https-enabled development server.
If you need a quick way to deploy your widget, you can use Netlify Drop.
Creating a Ticket Details widget
When developing an app in the Details section, you can add some content to the Opening state and display it in the Opening state preview. This way, you turn it into a Ticket Details widget.
Let's define the terms we use in this document:
- Section - an element of Ticket Details. It contains a complete set of information grouped under a single widget.
- Component - a single line in a section. It can have one of the pre-defined formats and be filled with data from the app.
How to add a widget
To display a widget, we have introduced the Opening state, which allows you to add components to your application and see a live preview of what the app will look like in the Opening state preview.
The components you may add are:
To read more about the HelpDesk Widgets, please see our App Guides.
How to update a section
You can control your widget and change its components using the HelpDesk SDK. Please refer to the Modify the Customer Details widget.
Component types
Here's the list of all the components you can use to build the Ticket Details app.
Header
Header is a container for components.
Example of a header component
{
"title": "card with image",
"components": [],
"imgUrl": "https://www.gstatic.com/webp/gallery/4.jpg",
"openApp": true
}
Property | Required | Type |
---|---|---|
title | Yes | string |
components | Yes | array of components |
imgUrl | No | string |
openApp | No | boolean |
Button
Simple button component
Example of a button component
{
"type": "button",
"data": {
"id": "second-button",
"label": "second button",
"openApp": true,
"primary": true
}
}
Property | Required | Type | Description |
---|---|---|---|
id | Yes | string | |
label | Yes | string | |
openApp | No | boolean | Default value: false |
primary | No | boolean | Default value: false |
secondary | No | boolean | Default value: false |
Click events
You can listen for button clicks using the SDK. Note that buttonId
will be the same as the id
from the schema. If you want to capture a specific click, you need to make sure that the id
is unique across all definitions.
Label with value
Example of label with value component
{
"type": "label_value",
"data": {
"label": "Name",
"value": "Stefan",
"iconUrl": "https://www.gstatic.com/webp/gallery/4.jpg"
}
}
Property | Required | Type | Description |
---|---|---|---|
label | No | string | |
value | No | string | |
iconUrl | No | string | |
url | No | string |
Link
Example of a link component
{
"type": "link",
"data": {
"value": "click me",
"url": "http://google.com",
"inline": false
}
}
Property | Required | Type | Description |
---|---|---|---|
url | Yes | string | |
value | No | string | |
inline | No | boolean | default: true |
Divider
Divider could be used to separate section content. It has no components inside.
Example of a divider component
{
"type": "divider"
}
Troubleshooting
There are errors in the console
Check out your browser's console to see if there are any of the errors listed below.
Error | Explanation |
---|---|
Refused to display '...' in a frame because an ancestor violates the following Content Security Policy directive: "...". | The host that serves the plugin has specific Content Security Policy set up. |
Refused to display '...' in a frame because it set 'X-Frame-Options' to 'SAMEORIGIN'. | The host serving the content of the plugin has specific X-Frame-Options header set up. |
Contact us
If you have any feature requests related to the App Widgets, let us know! We're open to your insights and suggestions. Feel free to drop us an email at developers@text.com or join our community on Discord and ask us your questions there.