Agent Authorization
Introduction
The authorization methods described in this document may be used to work with both LiveChat and HelpDesk products. You need to authorize your calls with an access token to call the APIs of any of these two products. This document describes three authorization flows that let you acquire a token:
- Personal Access Token
- Implicit flow
- Authorization code grant
With the acquired token, you can the following APIs on behalf of an agent:
- the Agent Chat API
- the Configuration API
- LiveChat Accounts API
- HelpDesk API
- Reports API
- Text API
To make calls to Customer Chat API, you need a different access_token
. For that, see Customer Authorization.
Postman collection
You can find all the requests from the Agent and Customer authorization flows in Postman. Remember to replace sample parameters with your own.
Personal Access Tokens
Unlike other authorization flows that let you acquire a token for your app, Personal Access Tokens (PATs) are generated per Agent. PATs are great when you want to make a quick call to the LiveChat API or test out the app you're working on.
To sign a request with a Personal Access Token, you need to:
- Create a PAT in Developer Console.
- Use your
account_id
and PAT in the Basic authentication scheme to send a request.
Step 1: Generate a PAT
Start in Developer Console. Then, go to Settings > Authorization > Personal Access Tokens and create a new token together with necessary scopes. You won't be able to change those scopes once you create the token.
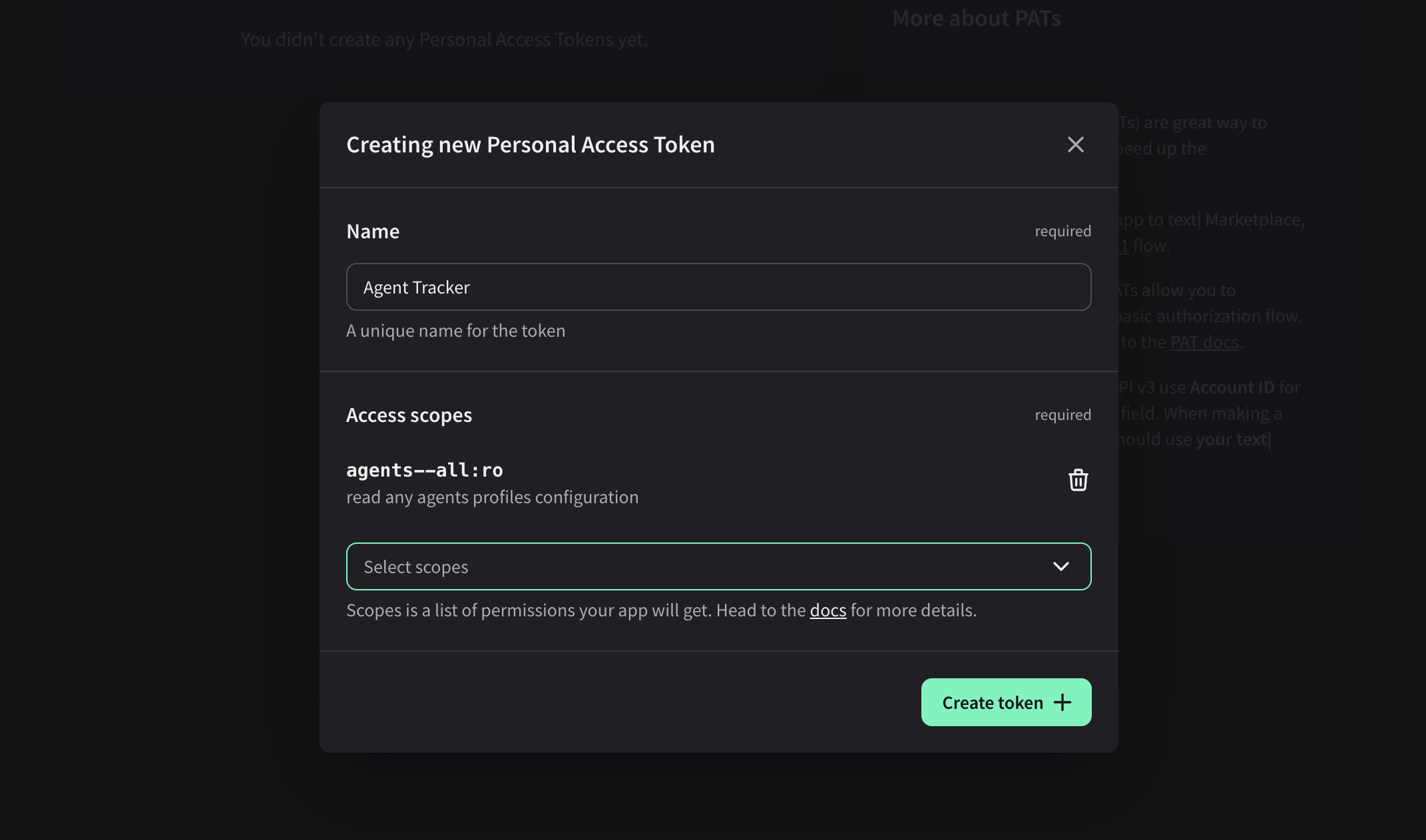
Step 2: Use your credentials to send the request
Personal Access Tokens use the Basic authentication scheme. For that reason, you need a username and a password. Please note that with Basic Auth, you need to encode your credentials using base64.
Use:
- Account ID as the username for the Agent Chat API v3, Configuration API v3, Global Accounts API, and HelpDesk API
- Entity ID (your LiveChat login) as the username for the Configuration API v2 (the deprecated version)
- PAT as the password for the APIs v2 (the deprecated version) and v3
Once you have your credentials, you can call the Agent Chat API, Configuration API, LiveChat Accounts API, or HelpDesk API. Other LiveChat APIs don't support PAT authorization.
Implicit grant
Implicit grant is an authorization flow recommended for JavaScript web apps, but it works for both types: JavaScript and server-side apps.
To set up your own web app, you must define the URL of the app and the list of scopes. Scopes determine which parts of a LiveChat user's account your app will have access to. A LiveChat customer who enters your app URL will be asked to enter their login and password and grant the access for your app. Then, the user is redirected to your app with access_token
included in the URL.
There are a few steps in the process:
- Step 1: Create an app in Developer Console and configure the Authorization building block
- Step 2: Redirect the users of your app to the LiveChat OAuth Server
- Step 3: Get an access token from the URL
- Step 4: Use the token in API calls
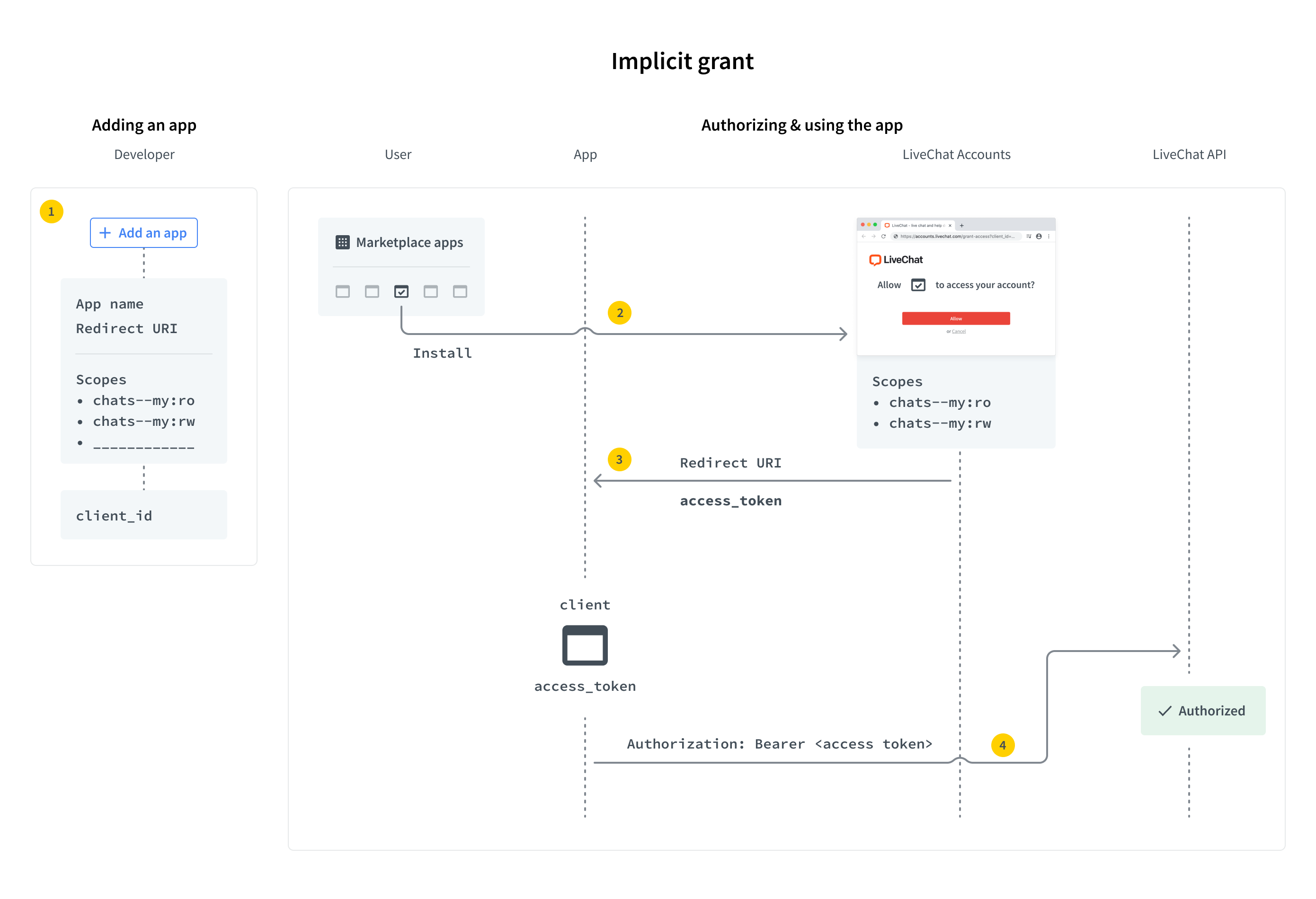
Step 1: Create an app
If you don't have an app yet, start by creating one in Developer Console. The app is a LiveChat OAuth 2.1 Client with its Id and Secret. You need to configure the Authorization building block of your application. See the guide to learn how to do that. Since this is the Implicit grant authorization flow, you won't use the Client Secret of your app. Feel free to skip the part about Client Secret in the guide; the rest applies without any change.
Step 2: Redirect users to LiveChat OAuth Server
When users run your app, they should be redirected to the LiveChat OAuth Server, which can be found under this URL:
LIVECHAT OAUTH SERVER URL
https:/
Request
Parameter | Required | Description |
---|---|---|
response_type | yes | Value: token |
client_id | yes | Client Id from Developer Console (Authorization block) |
redirect_uri | yes | One of the URIs defined in the Authorization block during app configuration. The LiveChat OAuth Server will redirect the user back to this URI after successful authorization. |
state | no | Any value that might be useful to your application. It's strongly recommended to include an anti-forgery token to mitigate the cross-site request forgery. |
prompt | no | Value: consent . For testing purposes. It forces the app to ask for access to certain resources. Itβs necessary for you to test the app as if you were a user who installs the app from Marketplace. |
EXAMPLE REDIRECTION TO LIVECHAT OAUTH SERVER
https://accounts.livechat.com/
?response_type=token
&client_id=9cbf3a968289727cb3cdfe83ab1d9836
&redirect_uri=https%3A%2F%2Fmy-application.com
&state=i8XNjC4b8KVok4uw5RftR38Wgp2BFwql
At this point, the app should ask the user to allow it to access certain resources and perform certain actions. The list of resources and actions is automatically created based on the scopes selected for your app in Developer Console.
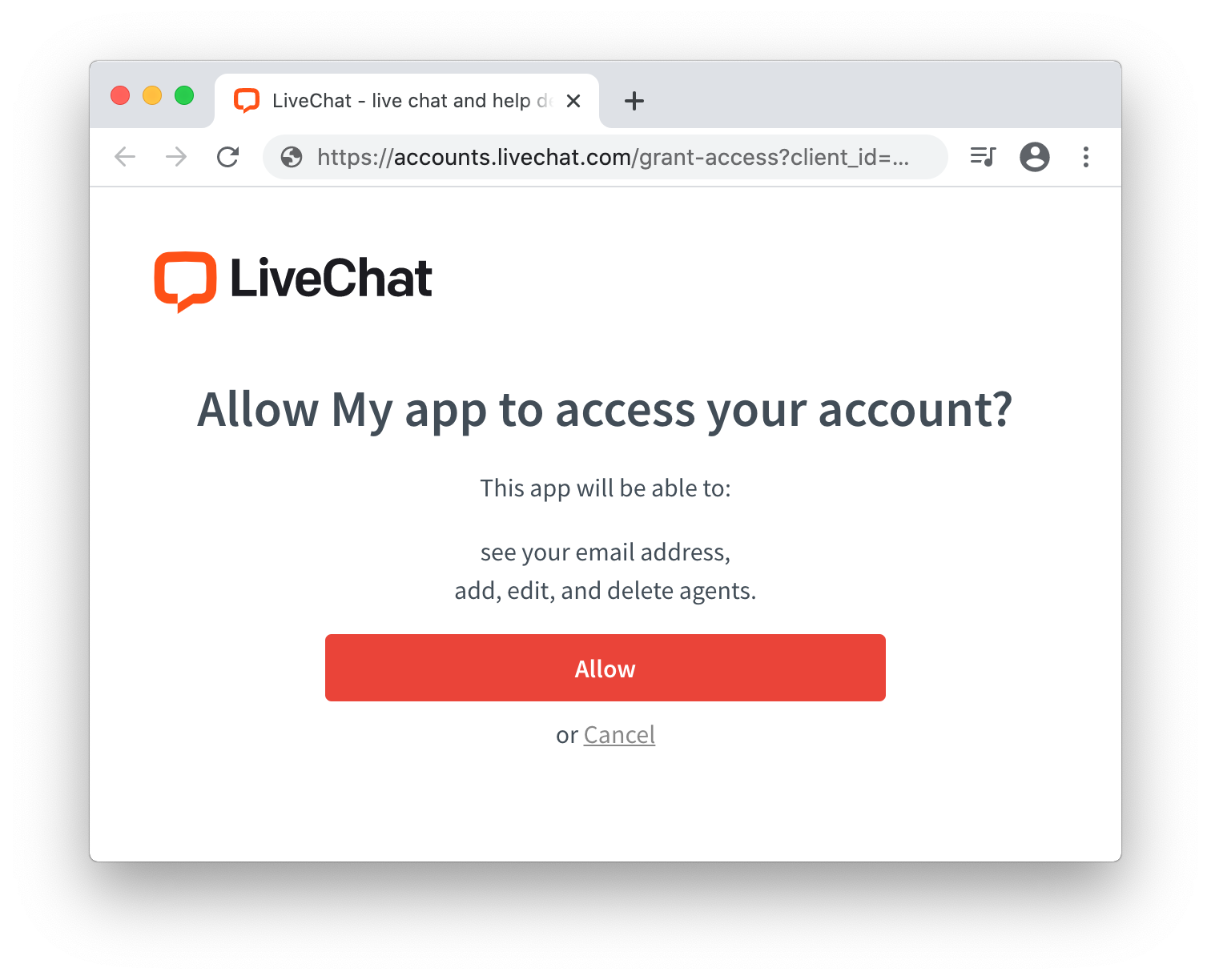
Keep in mind that as the app author, you won't see this screen. Use prompt:consent
to verify this step from the user perspective.
This step is also omitted for private web apps installed by Agents from the same license.
Step 3: Get an access token from the URL
After a user authorizes the app by clicking Allow, they are redirected back to your application (to the Redirect URI you specified in Developer Console). The URL includes a number of parameters, including the access_token
.
Response
Parameter | Description |
---|---|
access_token | The token you can use to call LiveChat APIs on behalf of the user. |
expires_in | The number of seconds the access_token will be valid; 28800 sec by default. When it expires, you will need to repeat the authorization process to get the new access_token . |
token_type | Value: Bearer |
state | The value of the state param that you passed to LiveChat OAuth Server in redirection. |
π‘ Got stuck? See common Errors.
EXAMPLE REDIRECTION BACK TO THE APP
https://my-application.com/
#access_token=dal%3Atest_DQTRHGbZCFkAoss4Q
&token_type=Bearer
&expires_in=28800
&state=i8XNjC4b8KVok4uw5RftR38Wgp2BFwql
Step 4: Use the token in API calls
Once you extract the token from the URL, your app can use it to sign requests to the LiveChat API.
Your application should store the access_token
in localStorage or a cookie until it expires. Caching the token prevents you from redirecting the user to LiveChat OAuth Server every time they visit your app.
Example implementation
This sample web app makes a call to Agent Chat API to return the list of customers, which is then logged in the console. The application uses the Implicit grant to get an access token.
index.html
<!DOCTYPE html>
<html>
<body>
<script src="/get_customers.js"></script>
β
<script>
function getHashParam(key) {
var params = location.hash.substring(1).split("&");
var value = params.find(function (item) {
return item.split("=")[0] === key;
});
return value ? value.split("=")[1] : "";
}
const clientId = "bb9e5b2f1ab480e4a715977b7b1b4279"; // Client Id of your app
const redirectUri = "https://get-customers-app.samplehosting.com/"; // URL of your app
const accessToken = decodeURIComponent(getHashParam("access_token"));
if (accessToken) {
get_customers();
} else {
location.href =
"https://accounts.livechat.com/" +
"?response_type=token" +
"&client_id=" +
clientId +
"&redirect_uri=" +
redirectUri;
}
</script>
</body>
</html>
get_customers.js
const get_customers = () => {
fetch('https://api.livechatinc.com/v3.1/agent/action/get_customers', {
method: 'post',
headers: {
'Content-type': 'application/json',
'Authorization': 'Bearer ' + accessToken,
},
body: JSON.stringify({})
})
.then((response) => response.json())
.then((data) => {
console.log('Request succeeded with JSON response', data);
})
.catch((error) => {
console.log('Request failed', error);
});
}
To make it work, run this app on localhost or deploy it to Firebase to host it. Update index.html
with your own redirectUri
(link to your app) and clientId
.
Make sure to use the exact same Redirect URI in Developer Console. Also, to use the Get Customers method, your app needs the customers:ro
scope, which you should select in Developer Console.
When everything is ready, install the app privately for your license.
Authorization code grant
Authorization code grant flow is recommended for server-side apps. Unlike web apps, they can store confidential info, such as Client Secret, on a server without ever exposing it. When a user runs your app, they are redirected to the LiveChat OAuth Server only once. After successful authorization, the user is redirected back to your app along with a single-use authorization code. Then, your application exchanges the code for an access token and a refresh token using the Client Secret. From now on, the app can regenerate new access tokens without any action required from the user.
This flow is very similar to Implicit grant, but contains one additional step of exchanging the code for an access token.
The steps are as follows:
- Step 1: Create an app in Developer Console and configure the Authorization building block
- Step 2: Redirect the users of your app to the LiveChat OAuth Server
- Step 3: Get a code from the URL
- Step 4: Exchange the code for an access token
- Step 5: Use the token in API calls
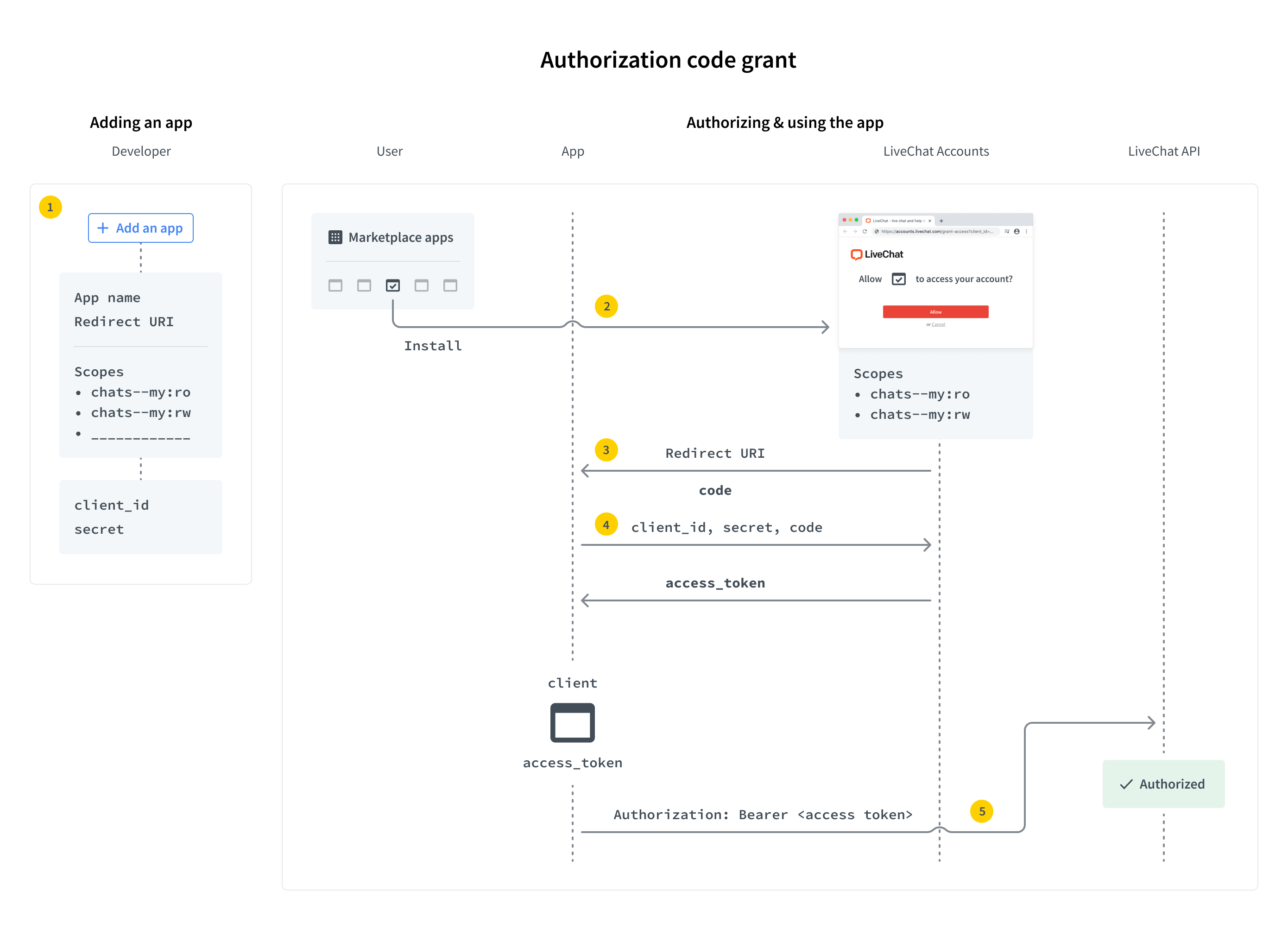
PKCE extension
OAuth 2.1 introduces the PKCE (Proof Key for Code Exchange) extension for the Authorization code grant flow.
It allows web applications to use the Authorization code grant flow, and also, enables the possibility to use custom schema redirects, like: my_app: //
(especially useful with native applications).
The Authorization code grant flow with PKCE is recommended for both web apps and server-side apps. Since web app clients can't store Client Secrets securely, their Authorization code grant flow with PKCE differs from the one for server-side apps.
- Web apps - Client Secret cannot be used, so it's not mandatory; refresh tokens rotate.
- Server-side apps - Client Secret is mandatory to exchange a code for an access token and to refresh a token; refresh tokens don't rotate.
How does the Authorization code grant flow work with PKCE?
- The client generates
code_verifier
from the following characters:[A-Z] / [a-z] / [0-9] / "-" / "." / "_" / "~"
. It's between 43 and 128 characters long. - The client generates
code_challenge
withcode_challenge_method
as follows:plain
-code_challenge
=code_verifier
, when nocode_challenge_method
is present, thenplain
is assumed.S256
-code_challenge
=b64(s256(ascii(code_verifier)))
whereb64
is Base64 URL encoding ands256
is theSHA256
hash function.
- The client sends
code_challenge
in the authorization request. - The server responds with the code.
- The client sends
code_verifier
during the exchane of the for an access token. - The server performs an additional validation for
code_challenge
andcode_verifier
. Upon successful validation, it returns the access token.
π‘ To see code samples with PKCE parameters, use the dropdown list.
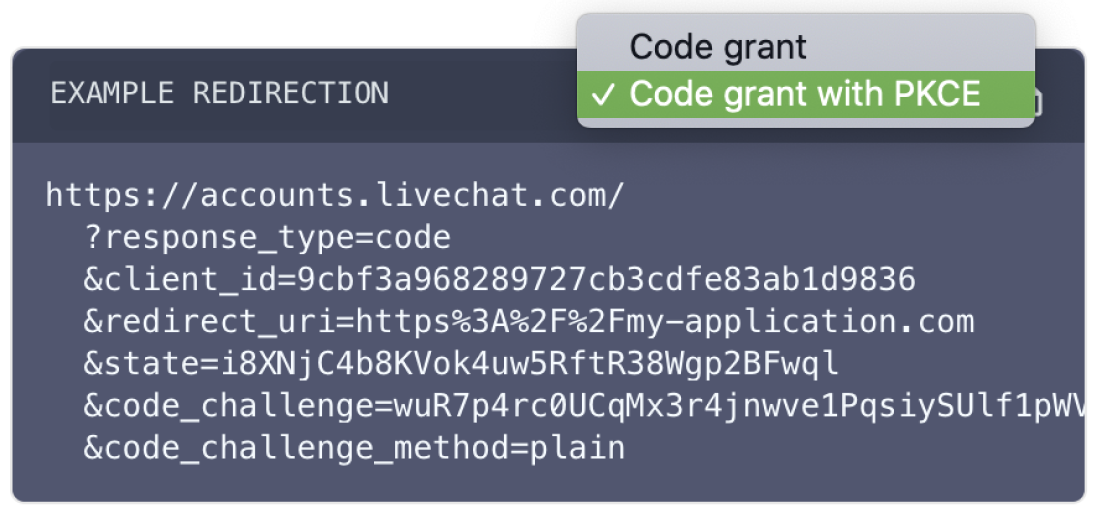
Step 1: Create an app
If you don't have an app yet, start by creating one in Developer Console. The app is a LiveChat OAuth 2.1 Client with its Id and Secret. You need to configure the Authorization building block of your application. See the guide to learn how to do that.
Step 2: Redirect users to LiveChat OAuth Server
When users run your app, they should be redirected to the LiveChat OAuth Server, which can be found under this URL:
EXAMPLE REDIRECTION TO LIVECHAT OAUTH SERVER
https:/
Request
Parameter | Required | Required with PKCE | Description |
---|---|---|---|
response_type | yes | yes | Value: code |
client_id | yes | yes | Client Id from Developer Console (Authorization block) |
redirect_uri | yes | yes | redirect_uri should be the same as Direct installation URL defined in the Authorization block during app configuration. The LiveChat OAuth Server will redirect the user back to this URI after successful authorization. |
state | no | no | Any value that might be useful to your application. It's strongly recommended to include an anti-forgery token to mitigate the cross-site request forgery. |
code_challenge | no | yes | A string between 43 and 128 characters long. |
code_challenge_method | - | no | Possible values: s256 or plain ; default: plain . |
EXAMPLE REDIRECTION
https://accounts.livechat.com/
?response_type=code
&client_id=9cbf3a968289727cb3cdfe83ab1d9836
&redirect_uri=https%3A%2F%2Fmy-application.com
&state=i8XNjC4b8KVok4uw5RftR38Wgp2BFwql
At this point, the app should ask the user to allow it to access certain resources and perform certain actions. The list of resources and actions is automatically created based on the scopes selected for your app in Developer Console.
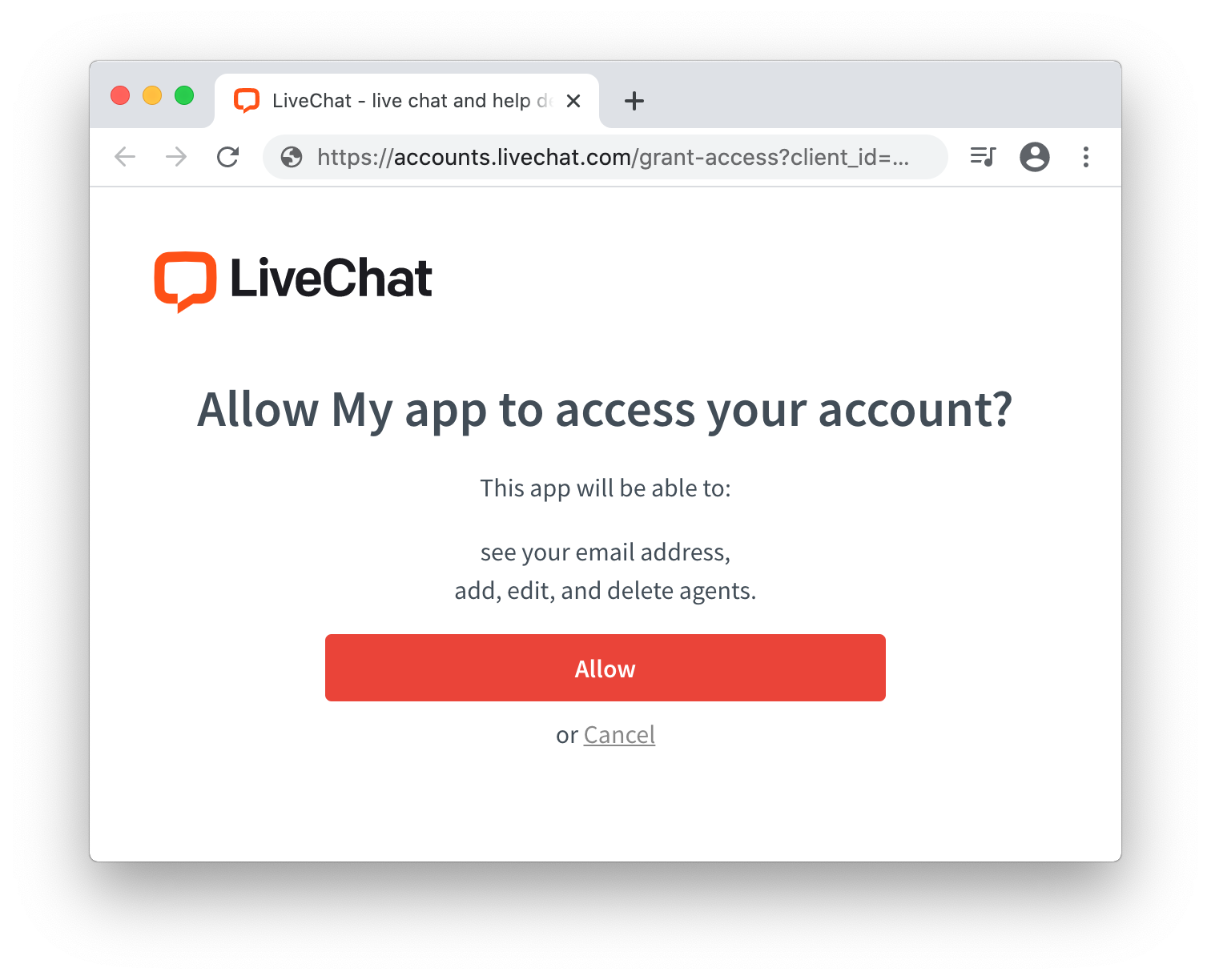
Keep in mind that as the app author, you won't see this screen. Use prompt:consent
to verify this step from the user perspective.
This step is also omitted for private server-side apps installed by Agents from the same license.
Step 3: Acquire the code
After a user authorizes the app by clicking Allow, they are redirected back to your application (to the Redirect URI you specified in Developer Console). The URL includes a number o parameters, including code
.
Request
Parameter | Notes |
---|---|
code | A single-use code you need to exchange it for an access token. It's only valid for a few minutes. |
state | The value of the state param that you passed to LiveChat OAuth Server in redirection. |
EXAMPLE REDIRECTION BACK TO THE APP
https://my-application.com/
?code=test_7W91a-oMsCeLvIaQm6bTrgtp7
&state=i8XNjC4b8KVok4uw5RftR38Wgp2BFwql
Step 4: Exchange code for access token and refresh token
To exchange the code
for an access_token
and a refresh_token
, you need to make an HTTP POST request to the following URL:
https://accounts.livechat.com/v2/token
Request
Parameter | Required | Required with PKCE server side app | Required with PKCE javascript app | Description |
---|---|---|---|---|
grant_type | yes | yes | yes | Value: authorization_code |
code | yes | yes | yes | The authorization code returned from the request in the previous step. |
client_id | yes | yes | yes | Client Id from Developer Console (Authorization block) |
client_secret | yes | yes | no | Unique, confidential identifier of your app from Developer Console (Authorization block). |
redirect_uri | yes | yes | yes | The URI defined in Step 2. The LiveChat OAuth Server will redirect the user back to this URL after successful authorization. |
code_verifier | no | yes | yes | The previously generated code_verifier . |
Response
The response is a JSON with the following parameters:
Parameter | Description |
---|---|
access_token | A token you can use to call LiveChat APIs on behalf of the user. |
account_id | The ID of the Agent's account |
expires_in | A number in seconds specifying how long the access_token will be valid; 28800 sec by default. When it expires, you will need to generate a new access_token using refresh_token (read Using the refresh token for more details). |
organization_id | The ID of the organization's account. |
refresh_token | A token that can be used to generate new access tokens. |
scope | A comma-separated list of permissions an access_token has. |
token_type | Value: Bearer |
π‘ Got stuck? See common Errors.
EXCHANGE CODE FOR TOKEN
curl "https://accounts.livechat.com/v2/token" \
-X POST \
-d "grant_type=authorization_code&\
code=dal:test_tnlRmy73mg9eaFESA&\
client_id=9cbf3a968289727cb3cdfe83ab1d9836&\
client_secret=test_d7MEp1YYo3&\
redirect_uri=https://my-application.com"
{
"access_token": "dal:test_YTJQ6GDVgQf8kQDPw",
"account_id": "b7eff798-f8df-4364-8059-649c35c9ed0c",
"expires_in": 28800,
"organization_id": "390e44e6-f1e6-0368c-z6ddb-74g14508c2ex",
"refresh_token": "test_gfalskcakg2347o8326",
"scope": "chats--all:ro,chats--all:rw",
"token_type": "Bearer"
}
π‘ Note: Refresh tokens no longer include the dal:
or fra:
prefixes.
Step 5: Use the token in API calls
Once you extract the token from the URL, your app can use it to sign requests to the LiveChat API.
Your application should store the access_token
in localStorage or a cookie until it expires. Caching the token prevents you from redirecting the user to LiveChat OAuth Server every time they visit your app.
Using the refresh token
When an access_token
expires, your app needs to acquire a new one. To do that, it has to send an HTTP POST request using the refresh_token
.
https:/v2/token
Request
Parameter | Required | Required with PKCE server side app | Required with PKCE javascript app | Description |
---|---|---|---|---|
grant_type | yes | yes | yes | Value: refresh_token |
refresh_token | yes | yes | yes | The refresh token returned from when exchanging the code . |
client_id | yes | yes | yes | Client Id from Developer Console (Authorization block) |
client_secret | yes | yes | no | Unique, confidential identifier of your app from Developer Console (Authorization block). |
Response
The response is a JSON with the following parameters:
Parameter | Description |
---|---|
access_token | A token you can use to call LiveChat APIs on behalf of the user. |
account_id | The ID of the Agent's account |
expires_in | A number in seconds specifying how long the access_token will be valid. When it expires, you will need to generate a new access_token using refresh_token (read Using the refresh token for more details). |
organization_id | the ID of the organization's account. |
scope | A comma-separated list of permissions an access_token has. |
refresh_token | A token that can be used to generate new access tokens. |
token_type | Value: Bearer |
π‘ Got stuck? See common Errors.
GET A NEW ACCESS TOKEN
curl "https://accounts.livechat.com/v2/token" \
-X POST \
-d "grant_type=refresh_token&\
refresh_token=test_gfalskcakg2347o8326&\
client_id=9cbf3a968289727cb3cdfe83ab1d9836&\
client_secret=test_d7MEp1YYo3"
{
"access_token": "dal:test_YTJQ6GDVgQf8kQDPw",
"account_id": "b7eff798-f8df-4364-8059-649c35c9ed0c",
"expires_in": 28800,
"organization_id": "390e44e6-f1e6-0368c-z6ddb-74g14508c2ex",
"scope": "chats--all:ro,chats--all:rw",
"refresh_token": "test_gfalskcakg2347o8326",
"token_type": "Bearer"
}
π‘ Note: Refresh tokens no longer include the dal:
or fra:
prefixes.
Revoking tokens
In some cases, a user may wish to revoke the access (the token) given to your application. The token can be either an access token or a refresh token. If it's an access token with a corresponding refresh token, both tokens will be revoked. To revoke a token, make a DELETE HTTP request to the following URL:
https:/v2/token
Code snippets present two alternative ways of making the same request.
Request
Parameter | Required | Description |
---|---|---|
code | yes | The value of the access_token or the refresh_token to revoke |
π‘ Got stuck? See common Errors.
REVOKE A TOKEN
curl "https://accounts.livechat.com/v2/token"
-H "Authorization: Bearer <access_token|refresh token>"
-X DELETE
{}
Validating the access token
You can validate an access_token
by making a GET HTTP request to the following URL:
https:/v2/info
Please note that refresh tokens are not supported for direct validation. If an access token was obtained using a refresh token, the response will return both tokens.
Response
The response is a JSON with the following parameters:
Parameter | Description |
---|---|
access_token | A token you can use to call LiveChat APIs on behalf of the user. |
account_id | The ID of the Agent's account |
client_id | Client Id of your app |
expires_in | A number in seconds specifying how long the access_token will be valid. When it expires, you will need to generate a new access_token using refresh_token (read Using the refresh token for more details). |
organization_id | The ID of the organization's account. |
scope | A comma-separated list of permissions an access_token has. |
refresh_token | A token that can be used to generate new access tokens. Returned optionally. |
token_type | Value: Bearer |
π‘ Got stuck? See common Errors.
VALIDATE AN ACCESS TOKEN
curl "https://accounts.livechat.com/v2/info"
-H "Authorization: Bearer <access_token>"
{
"access_token": "dal:test_YTJQ6GDVgQf8kQDPw",
"account_id": "bbe8b147-d60e-46ac-a1e5-1e94b11ea6e1",
"client_id": "9cbf3a968289727cb3cdfe83ab1d9836",
"expires_in": 28725,
"organization_id": "390e44e6-f1e6-0368c-z6ddb-74g14508c2ex",
"scope": "chats--all:ro,chats--all:rw",
"token_type": "Bearer"
}
Errors
If you get stuck or any error appears, please go to common Errors.